Last Updated on: (senast uppdaterad på:)
A Java EE application should be designed with a structure that is easily understandable and makes a balanced use of Data Access Objects, Entities, REST controller, transactions and a database back end. To have the app hosted on WildFly 18.0.1 application server we would require a connection to the database on the server. Below are the basics for the preparation process in order to have a basic application run:
1.
Set up a database. In our example, we set up a database called “WorkSuggest1” and set up a table within the database called “Person”:
create database WorkSuggest1;
CREATE TABLE `person` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) ,
`email` varchar(255) DEFAULT NULL,
`phone` varchar(255) DEFAULT NULL,
`address` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
)
2.
When the database is set up, start preparing the WildFly database connector and the data source that will be used in the application. A CLI script can be very useful to have this done. The script below (written by instructors at IT Högskolan Göteborg) helps to set up the module, given that you have the MySQL connector, so keep reading past the script below for more details. The link to the database is also needed. Be sure that the MySQL Connector is in c:\Users folder and the connector version is the one you have.
module add --name=com.mysql --resources=~/mysql-connector-java-8.0.19.jar --dependencies=javax.api,javax.transaction.api /subsystem=datasources/jdbc-driver=mysql:add(driver-name="mysql",driver-module-name="com.mysql",driver-class-name=com.mysql.cj.jdbc.Driver) data-source add --jndi-name=java:/worksuggest1 --name=worksuggest1 \ --connection-url="jdbc:mysql://localhost:3306/worksuggest1?allowPublicKeyRetrieval=true&useSSL=false&useJDBCCompliantTimezoneShift=true&useLegacyDatetimeCode=false&serverTimezone=UTC" \ --driver-name=mysql --user-name=root --password=root --min-pool-size=2 --initial-pool-size=4 --max-pool-size=6 /subsystem=logging/file-handler=fh:add(level=INFO, file={"relative-to"=>"jboss.server.log.dir", "path"=>"worksuggest1.log"}, append=true, autoflush=true) /subsystem=logging/logger=com.mygrouptasks.worksuggest1:add(use-parent-handlers=false,handlers=["fh"])
Normally, a MySQL connector is found in form of a Jar file such as the one found at Jar-download.com, which will accompany a “module.xml” file.
To complete the set up, follow a good tutorial on how to set up MySQL database connection on WildFly application server, such as this one.
In general, be sure that you have the database connector, the required module file, and the link to the database set up correctly as they are required for your Java EE application to interact with the database. Some side notes while you are setting them up are:
- The connector and the module.xml file should be placed in a directory structure that you create under the “base” folder hierarchy in the WildFly 18 folder structure — more specifically in the folder: C:\wildfly-18.0.1.Final\modules\system\layers\base. Here you will create a “com” folder, add a “mysql” folder there, and in it create a “main” folder. In this main folder you place the mysql connector file as well as the module.xml file.
- The module.xml file could have content similar to the one below.
<module name="com.mysql" xmlns="urn:jboss:module:1.5">
<resources>
<resource-root path="mysql-connector-java-8.0.18.jar">
</resource-root></resources>
<dependencies>
<module name="javax.api">
<module name="javax.transaction.api">
</module></module></dependencies>
</module>
3.
Continue the set up of the database for use via the application server. Given that you will use the CLI script above, place the CLI script in the bin folder of the WildFly 18.0.1 folder, go to the bin folder, and run the script while the server is running. Assuming you are using Windows PowerShell it would be:
.\jboss-cli -c --file=worksuggest1.cli
4.
Upon receiving success message, proceed to setting up the IntelliJ project which should have a Maven.pom.xml file with dependencies as described in the below pom.xml file:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mygrouptasks.worksuggest1</groupId> <artifactId>worksuggest1</artifactId> <version>1.0-SNAPSHOT</version> <packaging>war</packaging> <name>worksuggest1 Maven Webapp</name> <description>Work Suggest Application</description> <dependencies> <dependency> <groupId>javax</groupId> <artifactId>javaee-api</artifactId> <version>8.0.1</version> <scope>provided</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>4.0.1</version> <scope>provided</scope> </dependency> <dependency> <groupId>org.wildfly</groupId> <artifactId>wildfly-feature-pack</artifactId> <version>18.0.0.Final</version> <type>pom</type> <scope>provided</scope> </dependency> <dependency> <groupId>javax.ws.rs</groupId> <artifactId>javax.ws.rs-api</artifactId> <version>2.1.1</version> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.10</version> <scope>provided</scope> </dependency> <dependency> <groupId>org.hibernate.javax.persistence</groupId> <artifactId>hibernate-jpa-2.1-api</artifactId> <version>1.0.2.Final</version> </dependency> <dependency> <groupId>org.jboss.spec.javax.ejb</groupId> <artifactId>jboss-ejb-api_3.2_spec</artifactId> <version>2.0.0.Final</version> <scope>provided</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.5.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.testcontainers</groupId> <artifactId>mysql</artifactId> <version>${testcontainers.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.testcontainers</groupId> <artifactId>testcontainers</artifactId> <version>${testcontainers.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.json/json --> <dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20190722</version> </dependency> </dependencies> <build> <finalName>worksuggest1</finalName> <sourceDirectory>src/main/java</sourceDirectory> <plugins> <plugin> <groupId>org.wildfly.plugins</groupId> <artifactId>wildfly-maven-plugin</artifactId> <version>2.0.1.Final</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>${maven.compiler.source}</source> <target>${maven.compiler.target}</target> <compilerArgument>-Xlint:unchecked</compilerArgument> </configuration> </plugin> </plugins> </build> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <testcontainers.version>1.12.2</testcontainers.version> <failOnMissingWebXml>false</failOnMissingWebXml> </properties> </project>
5.
The persistence.xml file can be as follows:
<?xml version="1.0" encoding="UTF-8"?> <persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd" version="2.0" xmlns="http://java.sun.com/xml/ns/persistence"> <persistence-unit name="worksuggest1" transaction-type="JTA"> <jta-data-source>java:/worksuggest1</jta-data-source> <properties> <property name="hibernate.archive.autodetection" value="class, hbm" /> <property name="hibernate.hbm2ddl.auto" value="update" /> <property name="hibernate.dialect" value="org.hibernate.dialect.MySQL8Dialect" /> <property name="hibernate.show_sql" value="true" /> <property name="openjpa.jdbc.SynchronizeMappings" value="buildSchema(ForeignKeys=true)" /> <property name="openjpa.Log" value="SQL=TRACE" /> <property name="openjpa.ConnectionFactoryProperties" value="printParameters=true" /> </properties> </persistence-unit> </persistence>
6.
A file structure such as the one shown below can help with the development of the project with respective Java EE concepts considered:
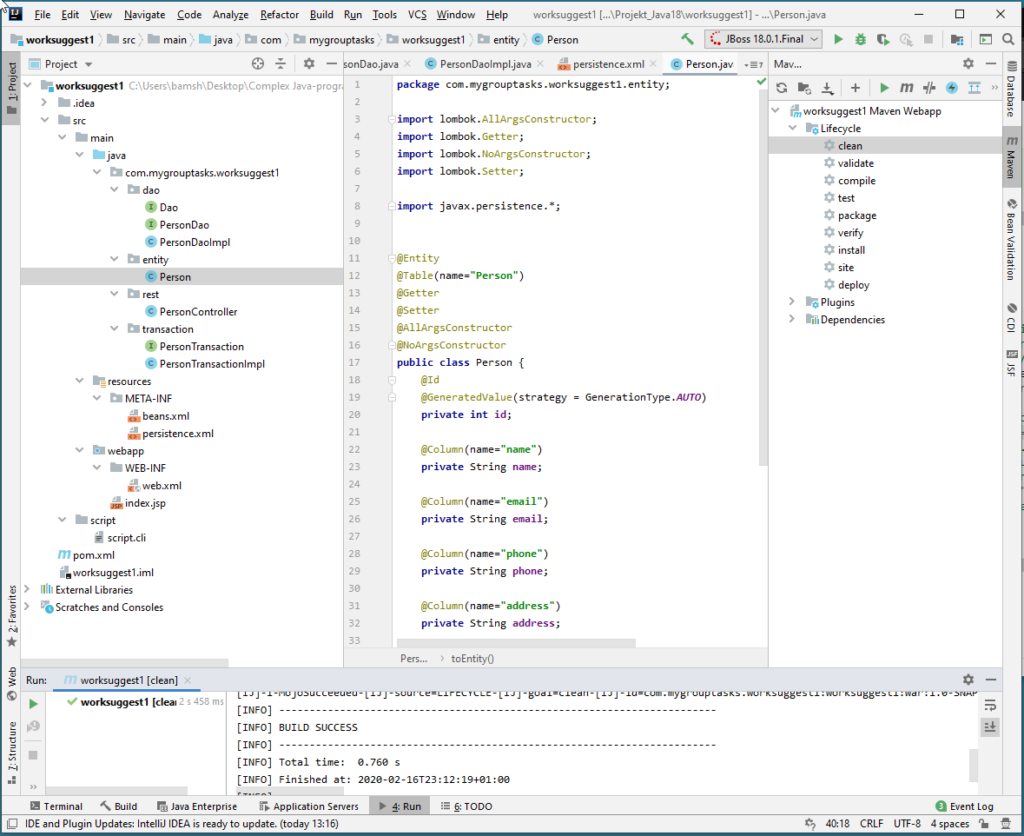
7.
The complete and working project (given that the MySQL database is available and the WildFly 18.0.1 application server are running) is included in the zip file below.